利用python绘制线型图
用法:
参数解释:
x,y
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | import numpy as np import matplotlib.pyplot as plt x = np.arange( 0.2 , 2.0 , 0.01 ) y1 = np.sin( 2 * np.pi * x) y2 = np.sin( 4 * np.pi * x) plt.figure( 1 ) plt.subplot( 211 ) plt.plot(x,y1) plt.subplot( 212 ) plt.plot(x,y2) plt.show() |
color
Colors的值:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import numpy as np import matplotlib.pyplot as plt # 需要解释下,下面两行代码是防止出现中文时,会报警告 # 因为我们的title里面写的是中文 plt.rcParams[ 'font.family' ] = 'SimHei' plt.rcParams[ 'axes.unicode_minus' ] = False x = np.arange( 0.2 , 2.0 , 0.01 ) y1 = np.sin( 2 * np.pi * x) y2 = np.sin( 4 * np.pi * x) plt.figure( 1 ) plt.subplot( 211 ) plt.title( '不添加颜色' ) plt.plot(x,y1) plt.subplot( 212 ) plt.title( '添加颜色' ) plt.plot(x,y2,color = 'c' ) plt.show() |
linstyle
1 2 3 4 5 | 'b' # blue markers with default shape 'or' # red circles '-g' # green solid line '--' # dashed line with default color '^k:' # black triangle_up markers connected by a dotted line |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | import numpy as np import matplotlib.pyplot as plt plt.figsize = (( 10 , 8 )) plt.rcParams[ 'font.family' ] = 'SimHei' plt.rcParams[ 'axes.unicode_minus' ] = False x = [ 1 , 2 , 3 , 4 ] y = [ 1 , 4 , 9 , 16 ] plt.subplot( 221 ) plt.title( '样式: -' ) plt.plot(x,y, '-' ) plt.subplot( 222 ) plt.title( '样式: --' ) plt.plot(x,y, '--' ) plt.subplot( 223 ) plt.title( '样式: -.' ) plt.plot(x, y, '-.' ) plt.subplot( 224 ) plt.title( '样式: :' ) plt.plot(x, y, ':' ) plt.show() |
缩写方式
1 2 3 4 5 6 7 8 | import numpy as np import matplotlib.pyplot as plt x = [ 1 , 2 , 3 , 4 ] y = [ 1 , 4 , 9 , 16 ] plt.subplot() # 线形状 '-',颜色'g' plt.plot(x, y, '-g' ) plt.show() |
marker, markersize
marker在scatter里面我已经有所解释过了,有好多种情况,可以在scatter散点图这里会将颜色和marker连接起来,可以有个很清楚的了解,并且较为清楚,也是缩写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import matplotlib.pyplot as plt plt.figsize = (( 12 , 6 )) plt.rcParams[ 'font.family' ] = 'SimHei' plt.rcParams[ 'axes.unicode_minus' ] = False x = [ 1 , 2 , 3 , 4 ] y = [ 1 , 4 , 9 , 16 ] plt.subplot( 131 ) plt.title( '默认情况' ) plt.plot(x, y) plt.subplot( 132 ) plt.title( '红色圆圈' ) # marker为o 颜色r plt.plot(x, y, 'or' ) plt.subplot( 133 ) plt.title( '正三角黑色' ) # marker为^ 颜色k->black plt.plot(x, y, '^k' ) plt.show() |
label
标签,这个在所有图形中都可以使用,在这里展示下,包括之前的alpha也是,都所属**kwargs里面,在任何绘图中都可以添加,legend为图例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | import matplotlib.pyplot as plt import numpy as np x = np.linspace( - np.pi / 2 , np.pi / 2 , 31 ) y = np.cos(x) * * 3 # 1) remove points where y > 0.7 x2 = x[y < = 0.7 ] y2 = y[y < = 0.7 ] # 2) mask points where y > 0.7 y3 = np.ma.masked_where(y > 0.7 , y) # 3) set to NaN where y > 0.7 y4 = y.copy() y4[y3 > 0.7 ] = np.nan plt.plot(x * 0.1 , y, 'o-' , color = 'lightgrey' , label = 'No mask' ) plt.plot(x2 * 0.4 , y2, 'o-' , label = 'Points removed' ) plt.plot(x * 0.7 , y3, 'o-' , label = 'Masked values' ) plt.plot(x * 1.0 , y4, 'o-' , label = 'NaN values' ) plt.legend() plt.show() |
下面就是一些案例
一次性绘制三个线条图
1 2 3 4 5 6 | import numpy as np import matplotlib.pyplot as plt t = np.arange( 0. , 5. , 0.2 ) # 红色虚线,蓝色方块,浅蓝六边形 plt.plot(t, t, 'r--' , t, t * * 2 , 'bs' , t, t * * 3 , 'cH' ) plt.show() |
1 2 3 4 5 6 7 8 9 10 11 | import numpy as np import matplotlib.pyplot as plt x1 = np.linspace( 0.0 , 5.0 ) y1 = np.cos( 2 * np.pi * x1) * np.exp( - x1) x2 = np.linspace( 0.0 , 2.0 ) y2 = np.cos( 2 * np.pi * x2) plt.subplot( 211 ) plt.plot(x1, y1, 'o-' ) plt.subplot( 212 ) plt.plot(x1, y1, '.-' ) plt.show() |
到此这篇关于利用python绘制线型图的文章就介绍到这了,更多相关python线型图内容请搜索脚本之家以前的文章或继续浏览下面的相关文章希望大家以后多多支持脚本之家!
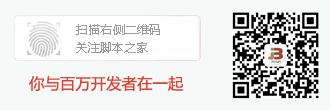
微信公众号搜索 “ 脚本之家 ” ,选择关注
程序猿的那些事、送书等活动等着你
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。
如若内容造成侵权/违法违规/事实不符,请将相关资料发送至 reterry123@163.com 进行投诉反馈,一经查实,立即处理!
相关文章
在Pycharm中将pyinstaller加入External Tools的方法
今天小编就为大家分享一篇在Pycharm中将pyinstaller加入External Tools的方法,具有很好的参考价值,希望对大家有所帮助。一起跟随小编过来看看吧2019-01-01
最新评论